OpenCLIPER classes
Overview
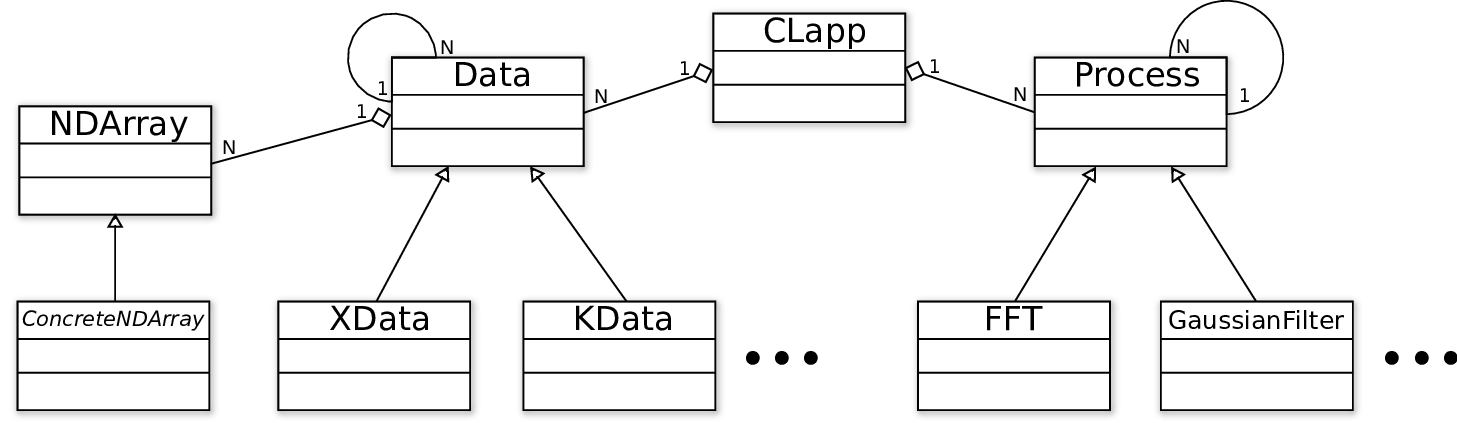
-
CLapp is the main class of OpenCLIPER. It acts as an interface to the OpenCL device and, as such, stores information about the current OpenCL platforms and devices, their associated command queues and so on. It also contains the list of data objects to be processed in the computing device. CLapp also deals with memory management of the computing device, as well as with data transfers to/from it. While OpenCLIPER is designed to minimize data transfers between the host and the processing device (since it is a common cause for major bottlenecks), it automatically uses pinned memory/buffer mapping in order to achieve maximum performance.
-
Data is an abstract class. Objects of its derived classes contain a set of images, volumes or n-dimensional data in the most general case. Each (derived from) Data object consists of one or more NDArray objects, which need not be equal in sizes or dimensions. This way, a single acquisition containing heterogeneous data may be stored in a single object. Data from an MRI scanner, for example, may contain K-space data, sensitivity maps, sampling masks, ECG signals, etc. Strides and positions for each element across any spatial or temporal dimension (row, column, slice, frame, etc.) are automatically calculated and made available to kernels in device memory space. Memory alignment is also dealt with, so that kernels can assume constant and predictable strides on every computing device.
-
Data is meant to be specialized for the problem to be solved, so final implementations may contain as many as necessary data types, be them real (such as for echo or radiograph data) or complex (such as for K-space data from an MRI scanner). OpenCLIPER provides two general-purpose specializations out-of-the-box called XData (for data with a direct physical interpretation) and KData (for K-space data). Readers and writers are provided for the most commonly-used image formats (via the OpenCV library), as well as for Matlab's .mat and CFL formats.
-
NDArray represents a signal, image, volume or any n-dimensional data structure. It is defined as an abstract class because it does not know the specific (machine) data type in which data are stored. Thus, it is limited to common attributes and methods for all possible data types, and to creation of objects of the actual container class, which is ConcreteNDArray. This way, implementers of new Data specializations do not have to deal with the deep details of data storage and class coupling is kept to a minimum.
-
ConcreteNDArray is a templated class which stores the actual data. It is not meant to be used by OpenCLIPER users but just to contain raw data and details which depend on the machine data type.
-
Process is an interface to algorithms which process data. As such, it is an abstract class which developers should derive from to implement their own processes. Its purpose is to provide a standard front-end to algorithms, so that no prior knowledge about their internals is needed to start working with them. Processes can be cascaded one after another incurring in no performance penalty (cascading is zero-copy).
Usually users of OpenCLIPER only have to deal with objects of CLapp, XData and KData classes and of subclasses of the Process class. Following sections show the main constructors and methods of these classes.
CLapp
Main CLapp methods:
- create: creates a new CLapp object and its list of devices that can be used as computing devices. Devices can be selected automatically, ordered by an estimated performance score, or according to the platform and/or device features specified by the user using the platformTraits and deviceTraits parameters. Overloaded versions of this method also allow to load a kernel or a list of kernels specified by their file names.
- loadKernels: load a kernel or list of kernels from a file or list of files.
- getContext: gets the OpenCL context associated to the platforms and devices available.
- getDevice: select one device from the list that contains the available devices that meet the platform and/or device selection criteria.
- getCommandQueue: gets the command queue associated to one device of the selected devices list.
- getProgram: get an OpenCL program from the list of loaded programs.
- getKernel: get an OpenCL kernel from the list of loaded kernels.
Data
Data is an abstract class used to derive XData and KData classes. Most useful Data methods (used by subclasses) are the following:
- getHostBuffer: gets a pointer to host memory where object data is stored.
- getDeviceBuffer: gets a pointer to a cl::Buffer object representing object data stored in device memory.
- getDataDimsAndStridesHostBuffer: gets a pointer to host memory where an array with information about spatial and temporal dimensions, number of coils (for KData objects) and related strides is stored.
- getDataDimsAndStridesDeviceBuffer: gets a pointer to a cl::Buffer object representing the array with dimensions and strides information.
- device2Host: copies data from device memory to host memory.
XData
XData constructors automatically create an XData object stored in host memory and add it to a previously created CLapp object (a mandatory constructor parameter), which copies all the needed data from host memory to device memory of the selected device. Main constructors let the user create an object with the following features:
- Empty object with specified spatial and temporal dimensions.
- Object with spatial and temporal dimensions (and optionally image content) copied from another XData or KData object.
- Image read from a file in an OpenCV library supported image format.
- Image(s) read from matlab format file.
- Image(s) read from raw format file(s).
The most commonly used methods in XData are:
- save: save one or several related images as a file or group of files in an image format supported by OpenCV library.
- matlabSave: save one or several related images as a matlab format file.
- saveRawHostData: save one or several related images as a file or group of files in raw format.
KData
KData constructors automatically create a KData object stored in host memory and add it to a previously created CLapp object (a mandatory constructor parameter), which copies all the needed data from host memory to device memory of the selected device. Main constructors let the user create an object with the following features:
- Empty object with specified spatial and temporal dimensions.
- Object with spatial and temporal dimensions (and optionally k-image, sensitivity maps and sampling masks data) copied from another KData object.
- Images read from matlab format file.
- Images read from raw format files.
The most commonly used methods in KData are:
- getSensitivityMapsData: gets pointer to SensitivityMapsData object containing sensitivity maps data (sensitivity values of the coil used to capture the image, one value per image pixel).
- getSamplingMasksData: gets pointer to SamplingMasksData object containing sampling masks data (sampling masks values are the list of line identifiers of the image lines acquired).
- multSensMaps: multiplies every image captured by a coil at some time by the coil sensitivity (a parameter lets conjugate the sensitivity map previously to the multiplication).
- xImageSum: adds all the images acquired by all the coils at the same time value and stores the result in an XData object (destination method parameter).
- matlabSave: save object content (k-images, sensitivity maps, sampling masks and array of dimensions and strides) as a matlab format file (sensitivity maps and sampling masks data are optional).
- saveRawHostData: save object content as a file or group of files in raw format.
Process
Process constructors automatically create a Process object and add it to a previously created CLapp object (a mandatory constructor parameter). Optionally, the input and output objects used for processing can be set. These objects must belong to a class derived from the Data class.
Process most used methods (most of them inherited from the ProcessCore class) are the following:
- setInput: sets the object subclass of Data used as source of data for processing.
- setOutput: sets the object subclass of Data used as destination of data for processing (for in-place calculations this object is the same as the object set as input).
- setInitParameters: sets the parameters needed for object initialization (usually set only once during the process life-cycle).
- setLaunchParameters: set the parameters needed for launching calculations (can be changed for every launch).
- init: initializes object (normally only once during the process life-cycle).
- launch: execute process calculations (using a kernel and data read from device memory, results are also stored in device memory).
Detailed documentation
OpenCLIPER class documentation is maintained with doxygen, here.